☰
Work together. Create smart machines. Serve society.
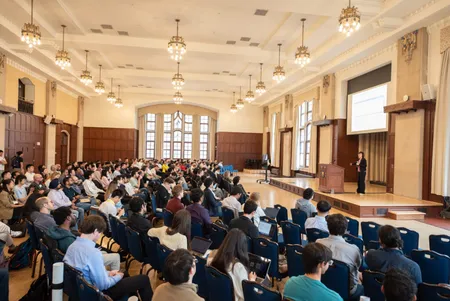
Michigan hosts 7th annual Learning for Dynamics & Control Conference
June 13, 2025
The conference attracted 300 people from around the world to discuss the latest cross-disciplinary approaches in this new scientific area.
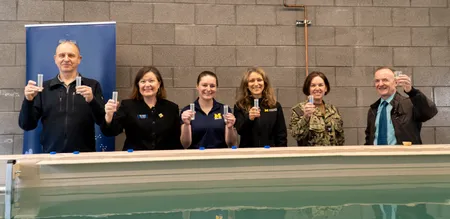
New robotics research water tank to enable advanced marine autonomy
May 7, 2025
A new 10,000 gallon water tank at the University of Michigan will help researchers design, build, and test a variety of autonomous underwater systems that could help robots map lakes and oceans and conduct inspections of ships and bridges.
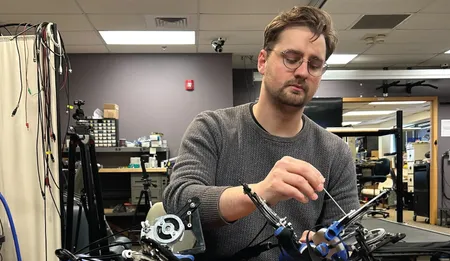
Low-cost technologies for stroke rehabilitation receive prize for outstanding PhD research
April 11, 2025
Thomas E Augenstein has been awarded the 2025 Towner Prize for Outstanding PhD Research. "The award recognizes Tom's extraordinary dedication and innovative contributions to stroke rehabilitation research over the past five years," said Dr. Chandramouli Krishnan.
Social media
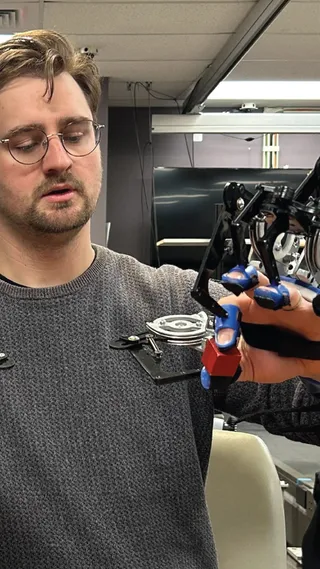
“The goal with these devices is really to get something that somebody can afford, or that a clinic can afford, so that we can really... help people who are currently not realizing their full recovery get there.” Thomas Augenstein received the @michi...
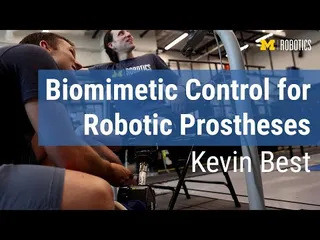
Kevin Best: Robotics PhD DefenseIn a PhD defense for Michigan Robotics, Kevin Best presents work to help advance robotic prostheses out of research labs and ...
A summary of what's been happening in the Michigan Robotics community, including: • the latest look at an effort to expand rural health care access • the role that gender of AI voices can affect our trust in autonomous vehicles • a lot more https://...